Terraform: Create a Virtual Cloud Network
In this tutorial, you use Terraform to create a virtual cloud network in your Oracle Cloud Infrastructure tenancy.
Key tasks include how to:
- Set up a basic virtual cloud network.
- Define and add the following resources to the network:
- Security lists
- Private and public subnets
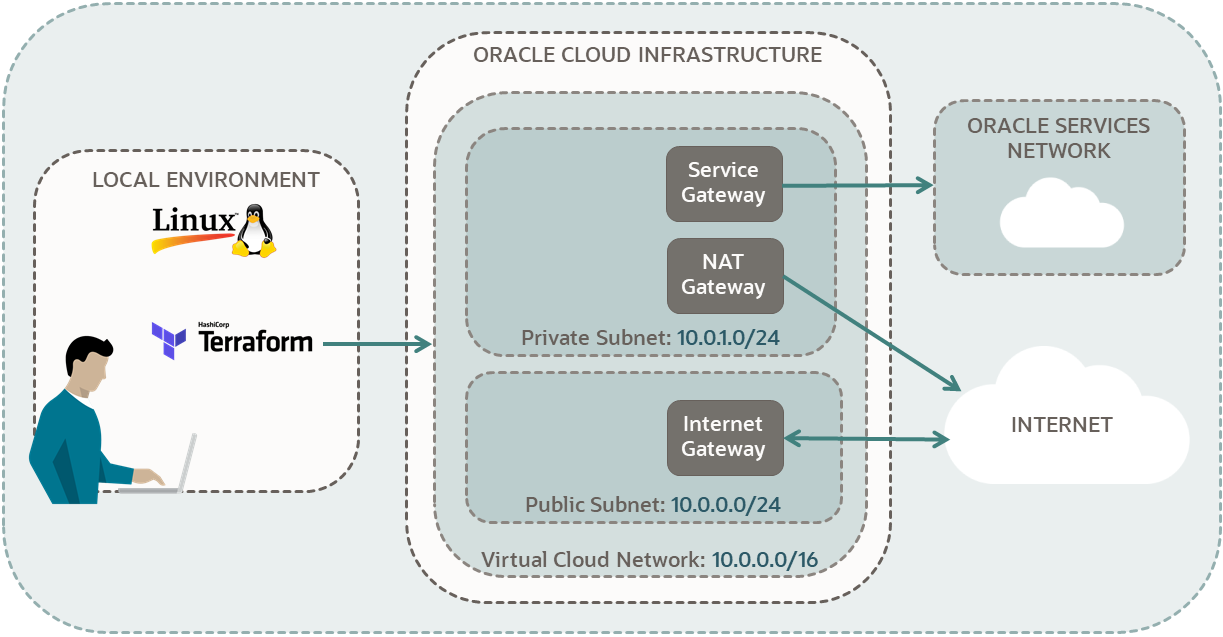
For additional information, see:
Before You Begin
To successfully perform this tutorial, you must have the following:
- A paid Oracle Cloud Infrastructure account. See Signing Up for Oracle Cloud Infrastructure.
- A MacOS, Linux, or Windows computer.
- Terraform tutorial resources:
- Go through all the steps in:
- Keep the scripts you created in the
following directories:
$HOME/tf-provider/
$HOME/tf-compartment/
- Keep the compartment from the Create a Compartment tutorial in your tenancy.
1. Prepare
Prepare your environment for creating a virtual cloud network. Also, collect all the information you need to complete the tutorial.
Copy the following information into your notepad.
If your username is in the Administrators group, then skip this section. Otherwise, have your administrator add the following policy to your tenancy:
allow group <the-group-your-username-belongs> to manage all-resources in compartment <your-compartment-name>
With this privilege, you can manage all resources in your compartment, essentially giving you administrative rights in that compartment.
- Open the navigation menu and click Identity & Security. Under Identity, click Policies.
- Select your compartment from the Compartment drop-down.
- Click Create Policy.
- Fill in the following information:
- Name:
manage-<your-compartment-name>-resources
- Description:
Allow users to list, create, update, and delete resources in <your-compartment-name>.
- Compartment:
<your-tenancy>(root)
- Name:
- For Policy Builder, select the following choices:
- Policy use cases:
Compartment Management
- Common policy templates:
Let compartment admins manage the compartment
- Groups:
<the-group-your-username-belongs>
- Location:
<your-tenancy>(root)
- Policy use cases:
- Click Create.
Reference: Common Policies
2. Create a Basic Network
Create scripts for authentication, a basic virtual cloud network defined by a module, and outputs.
First, set up a directory for your Terraform scripts. Then add a provider script so your Oracle Cloud Infrastructure account can authenticate the scripts running from this directory.
Declare a basic network with an Oracle Cloud Infrastructure virtual cloud network (VCN) module, documented in the Terraform Registry. Then, run your scripts and create the network. In the next sections, add components to customize your network.
About Modules
A module is a container for multiple resources that are used together. Instead of declaring infrastructure resources one by one, start with an Oracle Cloud Infrastructure provided module. For example, start with a basic virtual cloud network module. Then, add the resources that are not included in the module, to your scripts.
Declare a Module Block
- Start the block with the keyword:
module.
- Add a label for the module's provided name:
- Example:
"vcn"
- Example:
- Inside the code block
- Add source and version information from the Provision Instructions section of the module documentation.
- Provide a value for the required inputs. They don't have a
default value. Example:
- compartment_id
- internet_gateway_route_rules
- local_peering_gateways
- nat_gateway_route_rules
- region
- Provide values for the optional inputs that you want to override.
Otherwise, their default values are used. Example:
- create_internet_gateway
- create_nat_gateway
- create_service_gateway
- vcn_dns_label
- vcn_cidrs
- vcn_name
- You can declare the optional inputs with their default value, so later
when you review your code, you know what value you used.
Example:
vcn_name = "vcn-module" vcn_dns_label = "vcnmodule" vcn_cidrs = ["10.0.0.0/16"]
Add output blocks to your code to get information about your virtual cloud network after you run your scripts.
About Module Outputs
vcn
module:- Go to the OCI Module: vcn page.
- Click Outputs.
- You get a list of attributes that you can output for the VCN module.
- Review the description of the attributes:
- ig_route_id
- OCID of the route table that includes the internet gateway.
- nat_gateway_id
- OCID of the NAT gateway
- nat_route_id
- OCID of the route table that includes the NAT gateway.
- vcn_id
- OCID of the VCN
- ig_route_id
Declare a Module Output Block
-
- Start the block with the keyword:
output.
- Add a label to be printed with the output results:
- The label can contain letters, digits, underscores (_), and hyphens (-). The first character must not be a digit.
- Example:
"vcn_id"
- Get the attributes from the module's Outputs page.
- Inside the code block, enter a value for the module output with
the expression:
value = module.<module-name>.<output-attribute>
- Example:
value = module.vcn.vcn_id
- (Optional): Inside the code block, add a description string.
Example:
description = "OCID of the internet-route table. This route table has an internet gateway to be used for public subnets"
Note
A description string is not printed in the output, so ensure that the label describes what it outputs. - Create an output block for each output.
- Start the block with the keyword:
Congratulations! You have successfully created a basic virtual network using Terraform, in your Oracle Cloud Infrastructure account. You have a virtual network and you can be done at this point. The next sections show you how to customize a network created from a module.
3. Customize the Network
Create scripts for security lists, private, and public subnets to create the same virtual network as the Console's wizard.
- Go to Oracle Cloud Infrastructure Provider.
- In the left navigation Filter, enter
security list
.Results are returned for both Data Sources and Resources.
- Under Core, go to Resources and click oci_core_security_list.
- In the Argument Reference section, find all (Required) arguments
with top level (black solid) bullet points:
- compartment_id
- vcn_id
- For compartment_id:
use
compartment_id = "<compartment-ocid>"
- For vcn_id, use the OCID of the basic virtual network. To assign the
OCID, before knowing it, assign an output from the module, as input for
the security list resource:
- Get the module's output attribute from the module's Outputs page.
- Assign a value to the resource argument with the expression:
<resource argument> = module.<module-name>.<output-attribute>
- Example:
vcn_id = module.vcn.vcn_id
- Both the oci_core_security_list resource and the
oracle-terraform-modules/vcn use the same argument
name for virtual cloud network OCID:
vcn_id
. - The
vcn_id
on the left side of the equation is the argument (required input) for the resource. - The
vcn_id
on the right side of the equation, is the OCID of the VCN that you create with the module. - It doesn't matter if you have run the VCN module script and created the VCN or not. Either way, Terraform assigns the VCN OCID to the security list, after the VCN module is created.
Egress Rule
- Stateless: No
- Destination: 0.0.0.0/0
- IP Protocol: All Protocols
The Allows field in the table is automatically generated based on other fields. You don't add an argument for it in your script.
In the Argument Reference section of oci_core_security_list page, find the
following arguments for private-security-list.tf
:
- egress_security_rules
- stateless
- destination
- destination_type
- protocol
You use the equals sign "=" to assign a value to an argument. The argument
egress_security_rules
doesn't directly get a value. It has its own
arguments and they each get a value. Therefore, ensure that you only use the equals sign
inside the block.- Write:
egress_security_rules { <arguments with assigned values> }
- Don't write:
egress_security_rules = { <arguments with assigned values> }
In the Attribute Reference section of oci_core_security_list page, find the
following attributes to use as outputs in outputs.tf
:
- display_name
- id
Congratulations! You have successfully created a security list with an egress rule in your virtual cloud network. You add three ingress rules to this security list in the next section.
In this section, you add the following three ingress rules to the security list you created in the previous section.
Ingress Rules
- Rule 1:
- Stateless: No
- Source: 10.0.0.0/16
- IP Protocol: TCP
- Source Port Range: All
- Destination Port Range: 22
- Rule 2:
- Stateless: No
- Source: 0.0.0.0/0
- IP Protocol: ICMP
- Type and Code: 3, 4
- Rule 3:
- Stateless: No
- Source: 10.0.0.0/16
- IP Protocol: ICMP
- Type and Code: 3
The Allows field in the table is automatically generated based on other fields. You don't add an argument for it in your script.
Congratulations! You have successfully added three ingress rules to your security list. You use this security list for a private subnet. You create another security list for a public subnet in the next section.
- Go to Oracle Cloud Infrastructure Provider.
- In the left navigation Filter, enter
security list
.Results are returned for both Data Sources and Resources.
- Under Core, go to Resources and click oci_core_security_list.
- In the Argument Reference section, find the following arguments:
- ingress_security_rules
- stateless
- source
- source_type
- protocol
- icmp_options
- type
- code
- tcp_options
- min
- max
- ingress_security_rules
- For protocol, refer to Protocol Numbers:
- TCP: 6
- ICMP: 1
- For icmp_options, refer to ICMP Parameters.
- For tcp_options, if you have no port range, such as Destination Range:
22, set the maximum and minimum value to the same number. Example:
- min = 22
- max = 22
In this section, you create a security list in your network with egress and ingress rules. Later, you assign this security list to a public subnet.
Congratulations! You have successfully created another security list in your virtual cloud network.
In this section, you create a private subnet in your network and associate the private security list to this subnet. You also add the NAT route table that you made with the vcn module to this subnet. The NAT route table has one NAT gateway and one service gateway and is designed for private subnets. See the first diagram in the tutorial.
Congratulations! You have successfully created a private subnet in your virtual cloud network.
- Go to Oracle Cloud Infrastructure Provider.
- In the left navigation Filter, enter
subnet
.Results are returned for both Data Sources and Resources.
- Under Core, go to Resources and click oci_core_subnet.
- In the Argument Reference section, find all (Required) arguments:
- compartment_id
- vcn_id
- cidr_block
- Override the following optional arguments:
- route_table_id
- security_list_ids
- display_name
- Assign values to the arguments:
- cidr_block
- Refer to the first diagram in the tutorial.
- route_table_id
- The OCID of a route table.
- To see the gateways for this route table, refer to the private
subnet in the first diagram in the tutorial:
- NAT Gateway
- Service Gateway
- Assign the route table with the NAT gateway that you
created with the VCN module. This route table also contains a
service gateway.Note
- Use module.vcn.nat_route_id.
- Do not use module.vcn.nat_gateway_id, because it returns the OCID of the gateway and not the route table.
- (Optional): In the Console, go to
your-vcn-name
. Under Resources, click Route Tables and then nat-route. Review the rules of the route table and compare the Target Type values with the tutorial diagram.
- security_list_ids
- Returns a list of strings, each an OCID of a security list.
- Get the OCID of the private security list.
- Use square brackets for this argument. Example:
security_list_ids = ["sec-list-1","sec-list-2","sec-list-3"]
- To assign one security list, place it inside the square brackets without any commas.
- To refer to the security list created with another resource,
use its local name.
Example:
security_list_ids = [oci_core_security_list.<local-name>.id] security_list_ids = [oci_core_security_list.private-security-list.id]
- cidr_block
In this section, you create a public subnet in your network and associate the public security list to this subnet. You also add the internet route table that you made with the VCN module to this subnet. The internet route table has an internet gateway and is designed for public subnets. See the first diagram in the tutorial.
Congratulations! You have successfully created a public subnet in your virtual cloud network.
- Go to Oracle Cloud Infrastructure Provider.
- In the left navigation Filter, enter
subnet
.Results are returned for both Data Sources and Resources.
- Under Core, go to Resources and click oci_core_subnet.
- In the Argument Reference section, find all (Required) arguments:
- compartment_id
- vcn_id
- cidr_block
- Override the following optional arguments:
- route_table_id
- security_list_ids
- display_name
- Assign values to the arguments:
- cidr_block
- Refer to the first diagram in the tutorial.
- route_table_id
- The OCID of a route table.
- To see the gateway for this route table, refer to the public
subnet in the first diagram in the tutorial:
- Internet Gateway
- Assign the route table with an internet gateway that you
created with the VCN module.Note
- Use module.vcn.ig_route_id.
- (Optional): In the Console, go to
your-vcn-name
. Under Resources, click Route Tables and then internet-route. Review the rules of the route table and compare the Target Type value with the tutorial diagram.
- security_list_ids
- Returns a list of strings, each an OCID of a security list.
- Get the OCID of the public security list.
- Use square brackets for this argument. Example:
security_list_ids = ["sec-list-1","sec-list-2","sec-list-3"]
- To assign one security list, place it inside the square brackets without any commas.
- To refer to the security list created with another resource,
use its local name.
Example:
security_list_ids = [oci_core_security_list.<local-name>.id] security_list_ids = [oci_core_security_list.public-security-list.id]
- cidr_block
4. Recreate the Virtual Cloud Network (Optional)
Destroy your virtual cloud network. Then rerun your scripts to create another virtual cloud network.
In the previous sections, to check your work, you ran your scripts every time you declared a resource. Now, you run them together. You observe that the scripts are declarative and Terraform resolves the order in which it creates the objects.
Congratulations! You have successfully created a virtual cloud network and its components using Terraform, in your Oracle Cloud Infrastructure account.
This virtual cloud network has the same components as the virtual cloud network that you create in the Console, with the Start VCN Wizard. Choose the VCN with Internet Connectivity option. You can follow the tutorial steps to Set up a network with a wizard and then compare it with this network.
References:
What's Next
For the next Terraform: Get Started tutorial, go to:
To explore more information about development with Oracle products, check out these sites: